mirror of
https://github.com/teloxide/teloxide.git
synced 2024-12-23 06:51:01 +01:00
🤖 An elegant Telegram bots framework for Rust
https://docs.rs/teloxide
.github/workflows | ||
examples | ||
media | ||
src | ||
.gitignore | ||
Cargo.toml | ||
CODE_STYLE.md | ||
ICON.png | ||
LICENSE | ||
logo.svg | ||
README.md | ||
rustfmt.toml |
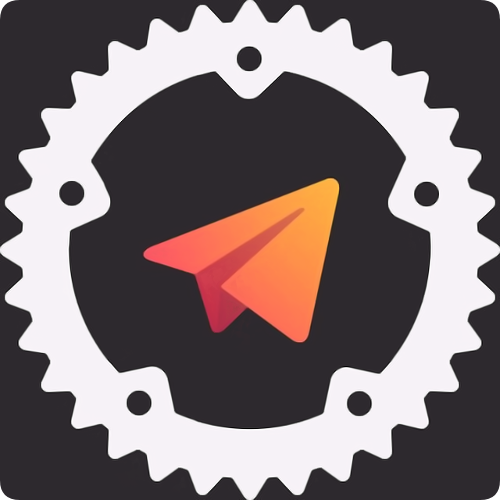
teloxide
A full-featured framework that empowers you to easily build Telegram bots using the async
/.await
syntax in Rust. It handles all the difficult stuff so you can focus only on your business logic.
Getting started
- Create a new bot using @Botfather to get a token in the format
123456789:blablabla
. - Be sure that you are up to date:
$ rustup update stable
- Execute
cargo new my_bot
, enter the directory and put these lines into yourCargo.toml
:
[dependencies]
teloxide = "0.1.0"
Writing your first bot
Open main.rs
file, because we're gonna write a ping-pong-bot!
use teloxide::prelude::*;
#[tokio::main]
async fn main() {
// Configure a fancy logger. Let this bot print everything, but restrict
// teloxide to only log errors.
std::env::set_var("RUST_LOG", "ping_pong_bot=trace");
std::env::set_var("RUST_LOG", "teloxide=error");
pretty_env_logger::init();
log::info!("Starting the ping-pong bot!");
// Creates a dispatcher of updates with the specified bot. Don't forget to
// replace `MyAwesomeToken` with yours.
Dispatcher::<RequestError>::new(Bot::new("MyAwesomeToken"))
// Registers a message handler. Inside a body of the closure, answer
// `"pong"` to an incoming message.
.message_handler(&|ctx: DispatcherHandlerCtx<Message>| async move {
ctx.answer("pong").send().await?;
Ok(())
})
.dispatch()
.await;
}