mirror of
https://github.com/teloxide/teloxide.git
synced 2024-12-31 16:40:37 +01:00
🤖 An elegant Telegram bots framework for Rust
https://docs.rs/teloxide
.github/workflows | ||
examples | ||
media | ||
src | ||
.gitignore | ||
Cargo.toml | ||
CODE_STYLE.md | ||
ICON.png | ||
LICENSE | ||
logo.svg | ||
README.md | ||
rustfmt.toml |
WARNING: this library is still in active development under v0.1.0, use it at your own risk!
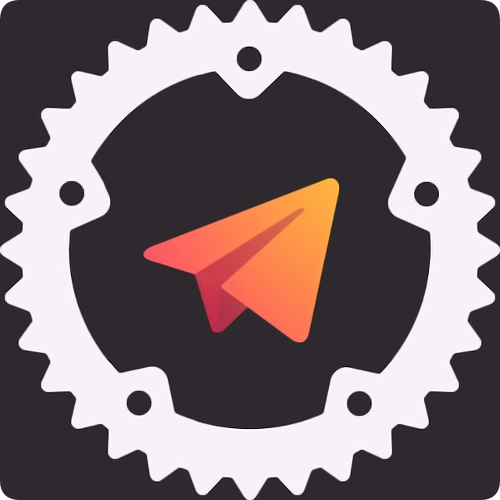
teloxide
A full-featured framework that empowers you to easily build Telegram bots using the async
/.await
syntax in Rust. It handles all the difficult stuff so you can focus only on your business logic.
Getting started
- Be sure that you are up to date:
$ rustup update stable
- To create a new bot, execute
cargo new
and put the following lines into yourCargo.toml
:
[dependencies]
teloxide = "0.1.0"
Writing first bot
First, create bot with @botfather. After creating, botfather give you
token in format 123456789:somemanyletters
.
Next, open yout main.rs
file. Let's create a simple echo bot:
use futures::stream::StreamExt;
use teloxide::{
dispatching::{
chat::{ChatUpdate, ChatUpdateKind, Dispatcher},
update_listeners::polling_default,
SessionState,
},
requests::Request,
Bot,
};
#[tokio::main]
async fn main() {
let bot = &Bot::new("1061598315:AAErEDodTsrqD3UxA_EvFyEfXbKA6DT25G0");
let mut updater = Box::pin(polling_default(bot));
let handler = |_, upd: ChatUpdate| async move {
if let ChatUpdateKind::Message(m) = upd.kind {
let msg = bot.send_message(m.chat.id, m.text);
msg.send().await.unwrap();
}
SessionState::Continue(())
};
let mut dp = Dispatcher::<'_, (), _>::new(handler);
println!("Starting the message handler.");
loop {
let u = updater.next().await.unwrap();
match u {
Err(e) => eprintln!("Error: {}", e),
Ok(u) => {
let _ = dp.dispatch(u).await;
}
}
}
}